What is Redux?
- Titash Roy
- Jul 25, 2020
- 3 min read
Updated: Jul 28, 2020
Introduction In previous blogs, we have discussed about the usefulness of store. In this blogs we will focus on the ways we can achieve that. One such way is REDUX. Redux is an open-source JavaScript library for managing application state. It is most commonly used with the view libraries such as React or Angular for building user interfaces. In Redux, we have a single store, which in contrast to FLUX (store developed by Facebook having multiple stores — out of context of this series), makes store management easier. To install redux and react with redux, we need the following commands: npm install –save redux npm install –save react-redux Redux-Data flow The components of redux has been covered in the last blog —https://titashrdomain.wordpress.com/2020/07/18/what-is-store-in-react/. Next we would discuss the data-flow in Redux. The data flow in redux is unidirectional. This makes it easier to extend the functionality in the code without having to worry of any unexpected behavior as the state is maintained in a single store. Below are the steps of data flow in redux: # An action is dispatched when a user does any activity in the application. # The root reducer is called with the current state and the dispatched action. The root reducer may be a combination of smaller multiple reducers to divide the task. # Inside the specific reducer the state is manipulated as per the action. # The store notifies the view by executing their callback functions. # The view can retrieve updated state and re-render again.

What is Provider? We saw how the store works and how it updates the state based on the dispatched action. But how to make the store available to all the required components? This is where Provider comes into picture. Since any React component in a React-Redux app can be connected to the Redux store, most applications will render a <Provider> at the top level, with the entire app’s component tree inside of it. Below is a snippet where the <Provider/> is wrapped over the main app. We mostly write the Provider and the create store in the main index.js file of the app.
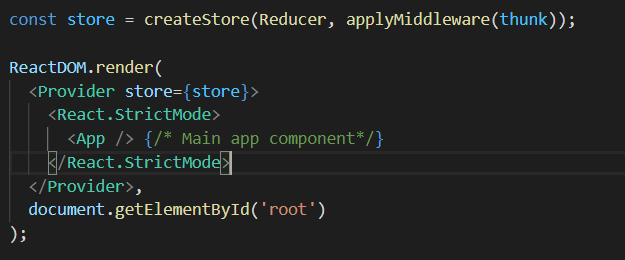
What is connect? Connect is a function that comes with the react-redux dependency. This function is used to connect the Redux store to the react components. NOTE: Even if the component is connected, if we don’t wrap it under the <Provide/>, store won’t be available to it. Connect() function can be thought of a HOC–>Higher-Order-Component (https://titashrdomain.wordpress.com/2020/06/20/what-are-higher-order-components-hoc-in-react/), which takes in the connected component as an input, manipulatesthe component as per the required parameters received and returns the updated component. The parameters which connect() function accepts as arguments are # mapStateToProps – This function takes at max of two arguments, one is store state and the other is ownProps. Based on these options, it modifies the component and returns updated component. # mapDispatchToProps – This parameter can be either a function or an object. It takes in a max of 2 parameters. One is the dispatch to store and the other is ownProps. # mergeProps – This parameter is a function and can be thought of a merging to the above two props. It takes in 3 parameters the results of mapStateToProps and mapDispatchToProps and the component’s ownProps. # options – React-Redux allows us to supply another parameter, which is a custom object to provide some additional characteristics to our updated component. This parameter has various attributes that change the behavior of the updated component accordingly. NOTE: We would discuss about each of these parameters individually in subsequent blogs. Below is a simple snippet of connect. The SecondChild is the wrapped component in the below snippet and the connect() is working as the HOC.
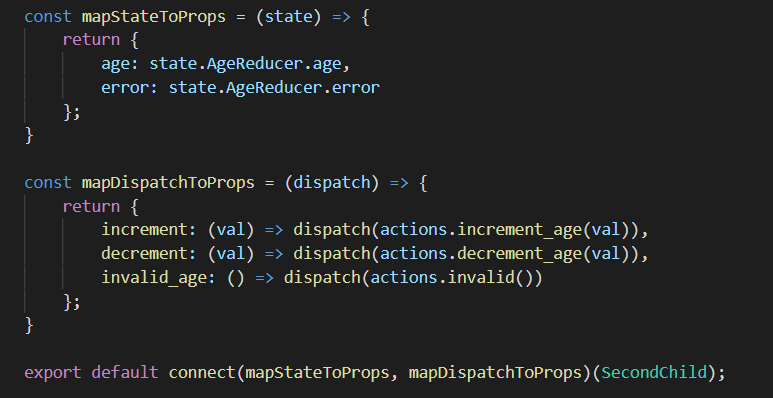
Summary In this blog, we have discussed about REDUX, the data flow in Redux, Provide and a brief introduction on connect() function.
Comments