Introduction As we have seen the functionality of Redux in previous posts, we know that its synchronous. So how does Redux handle asynchronous network calls to external API. This is where Redux middleware come into picture. It provides a third-party extension point between dispatching an action, and the moment it reaches the reducer. People use Redux middleware for logging, crash reporting, talking to an asynchronous API, routing, and more.
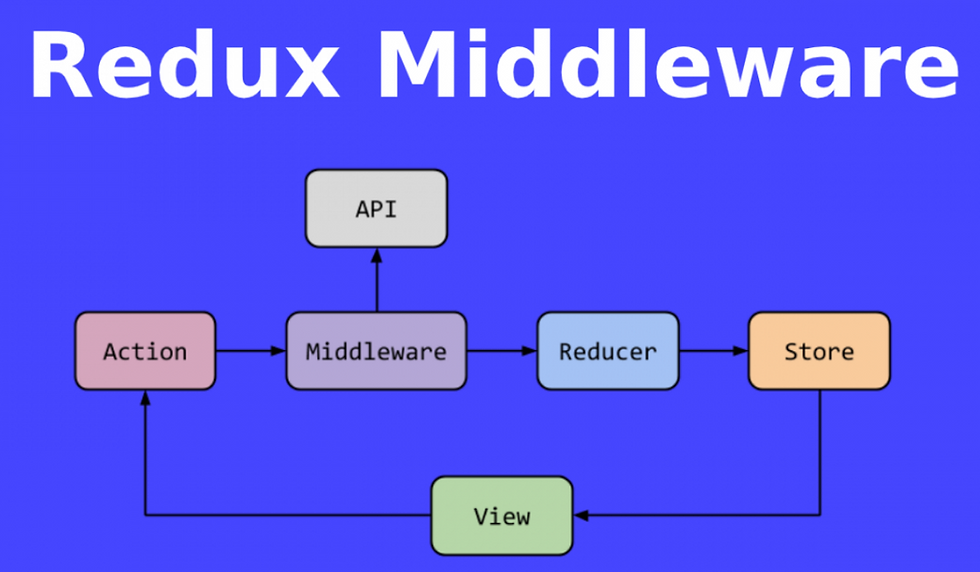
Where to introduce the middleware? Redux middle should be included while creating the store. It is done in the index.js file. Below in the code snippet.
import { createStore, applyMiddleware } from 'redux';
import Reducer from './reducers/index';
import thunk from "redux-thunk";
const store = createStore(Reducer, applyMiddleware(thunk));
In the above snippet, the middleware used is redux-thunk. We will discuss about the major types of redux middleware in the next section.
Types of middleware
Redux thunk - Redux Thunk middleware allows you to write action creators that return a function instead of an action. The thunk can be used to delay the dispatch of an action, or to dispatch only if a certain condition is met. The inner function receives the store methods, dispatch and action as parameters. We have seen how to include thunk in our store in the above section. Below is a snippet of Redux thunk in implementation. In the below snippet, the function get user is returning a function, which internally waits till the response from the https://randomuser.me/api/ is received. It takes the output of the API and passes it to the dispatch function, which in turn calls an action creator after certain timeout.
export function get_user_details(val) {
return {
type: userActionTypes.getUser,
payload: val
};
};
export function get_user() {
return (dispatch) => {
return axios.get(`https://randomuser.me/api/`)
.then(res => {
const person = res.data.results[0];
setTimeout(() => { dispatch(get_user_details(person)) }, 3000);
});
}
};
Redux saga - Redux saga is another kind of middleware used where we watch for an action being called. This watch function is a generator function [Generator function is a ES6 concept of JS, Generator functions allow you to define an iterative algorithm by writing a single function whose execution is not continuous. Generator functions are written using the function* syntax]. Once the watcher, finds the required action, it calls another action creator[generator function] that performs the async function or timeout. The generator functions have many inbuilt helper functions that is required for redux saga to work. NOTE: Generators are beyond the scope of this post. Below is a snippet. In the below snippet, AgeUp() is a generator function which watches for the action AGE_UP, which we dispatched from our component on an event. The yield keyword pauses generator function execution and the value of the expression following the yield keyword is returned to the generator's caller. In our case, the generator Function AgeUP() is halted till the ageUpAsync is executed, which in-turn waits for the delay(same as setTimeout). Once the delay is completed, the reduces listens to the action type: "AGE_UP_ASYNC" and performs the state update.
import{delay}from"redux-saga";
import{takeLatest,put}from"redux-saga/effects";
function* ageUpAsync() {
yield delay(4000);
yield put({ type: "AGE_UP_ASYNC", value: 1 });
}
export function* AgeUp() {
yield takeLatest("AGE_UP", ageUpAsync);
}
Below snippet shows how we should include redux saga in our application. The AgeUp() function in the import is the same AgeUp generator function shown in the above snippet. This function is passed as a parameter in the run method as shown in the snippet. NOTE: If we have several generator function(watcher functions), which corresponds to an action, where we want to use middleware, we need to include all under the run() as shown below.
import createSagaMiddleware from "redux-saga";
import { AgeUp } from "./sagas/saga";
const sagaMiddleware = createSagaMiddleware();
const store = createStore(reducer, applyMiddleware(sagaMiddleware));
sagaMiddleware.run(AgeUp);
Summary Redux middleware is advanced concept in Redux and should be used as per our needs if we want any asynchronous actions in our application. In this post we have discussed what is middleware and two of the post widely used middleware. Be careful with the syntax of both the types.
Comentarios