What are refs in React?
- Titash Roy
- Jun 28, 2020
- 2 min read
Updated: Jul 28, 2020
Introduction In the typical React data-flow, props are the only way for a parent component to interact with their children. In order to make any change in the child, we need to re-render it with new props. However, in some cases, we may want to modify some React component or DOM element in the child, without re-rendering the component with new props from the parent. This is where refs come into picture. When to use refs? There are a various reasons when we may need to use refs. Below are a few: 1. Managing focus, text selection or media playback 2. Using third-party libraries 3. Displaying animations NOTE: Avoid rigorous use of refs. We should avoid using refs while making more declarative usage We should make sure how we want our DOM element to perform before thinking of using refs. If we need the activity performed on the element or component to be visible to other components in the react data-flow, we should think of lifting state up to a parent component so that it is visible and available for other components as well. We should keep this condition in mind before using refs. In such a case, a better option would be to use state and pass it as props to a component. Using refs in class component In class components, refs can be created using React.createRef() function. Below is snippet.
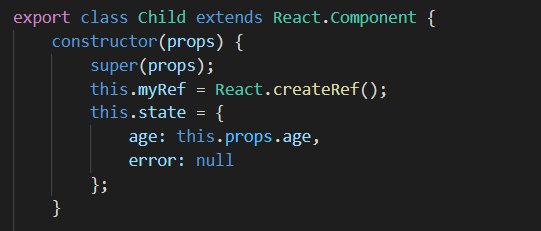
In the above snippet, a ref named as myRef is created. this can be used in the DOM element as follows.
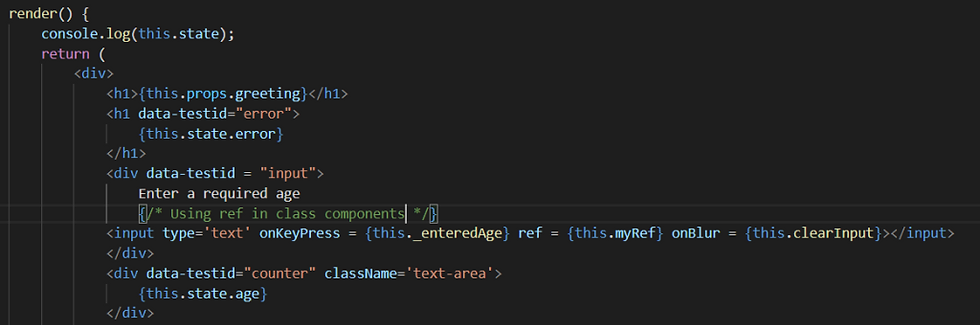
In the above snippet, we have an input box and we created a ref for it. We would use this ref to handle any change in the input box. In this we don’t interact or fetch the value from props. It is solely to the DOM element itself. The source code can be found here – https://github.com/Titashr/my_react_app Using refs in functional components Refs in functional component was not possible till the introduction of hooks as functional components didn’t have an instance. From react 16.8, we can use refs in react using useRef() hook. Below is a snippet.

Summary In this blog, we have discussed what are refs, the use and importance of refs. Creating refs in functional and class components.
Comments