How to use React Lifecycle methods to our advantage?
- Titash Roy
- Jun 13, 2020
- 3 min read
Updated: Jul 28, 2020
Introduction Hope you have read the previous blog having an introduction to the lifecycle methods. These methods are for the class component only. This post is mostly aimed at how and when to use the react lifecycle methods to get the best benefit out of them. NOTE: I will not be mentioning all of them in details, just the important points and their counterpart from react 16 onward Initialization phase Constructor – This is where we should initialize the default state of the component and also the extend the props from the parent React.Component, in case we want to use props in our class component. Below is the snippet.
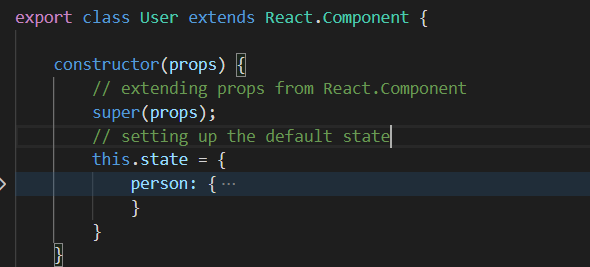
ComponentWillMount – This method is called before the initial render on both server and client side. From React 16, this has been replaced with getDerivedStateFromProps, which is a static method and as the name suggests, it takes the props from the parent and sets the state. This does a part of work of the constructor so this method is mostly ignored and the constructor is used instead. ComponentDidMount – This method is executed after the first render only on the client side. This is where we should make AJAX requests and DOM or state updates. This method is also used for integration with other JavaScript frameworks. Below is a snippet.

Updating Phase ComponentWillReceiveProps – This method is used to update the component state based on any prop change due to some REDUX store update or AJAX call. It is invoked as soon as the props are updated before another render is called. In React 16, this is also replaced with getDerivedStateFromProps. ShouldComponentUpdate – As said in the previous blogs, this methods is used to make the component behave as a pure-component, i.e., it won’t run the render() if there is no change in the state of the component. It returns the a boolean value and based on which the component is re-rendered. ComponentWillUpdate – This method is called once we have decided to update the component. It takes in nextProps and nextState as arguments. For accessing the old props or state, we can call this.props or this.state. We can then compare them to the new values and make changes/calculations as required before the render method is called. This method can be used for accessing Native UI and starting animation. Here we can also make property changes through dispatch(To be explained in the REDUX section) and AJAX calls. Unlike componentWillMount(), we should not call this.setState() here. The reason behind this is that the method triggers another componentWillUpdate() and eventually, we will end up in an infinite loop. In React 16, this has been changed to getSnapshotBeforeUpdate – It is a kind of screenshot of what the previous state and props look like before the component was updated. It takes in prevProps and prevState as arguments and perform calculations just like componentWillMount. It either returns a value from the conditional statement or null by default. The value returned is always passed down to the componentDidUpdate method. Below are some snippets.

ComponentDidUpdate – Called after every render() method, except the initial render method. Takes prevProps and prevState as argument. Can be used to perform state updates or external API calls, but conditionally to prevent unnecessary external calls. From React 16 onwards, an additional argument is added in this method, which is the snapshot received from getSnapshotBeforeUpdate. Below is the snippet of from react 16 onwards.
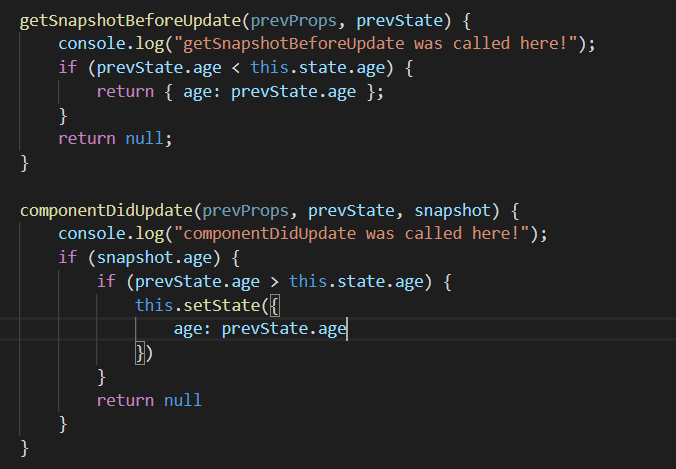
Error handling– ERROR BOUNDARY Two new lifecycle methods created to handle errors introduced in react 16. getDerivedStateFromError – If a child component of a parent component has an error we can use this method to display an error screen with an error message rather than a blank screen. Below is a snippet.

ComponentDidCatch – This is the second lifecycle method that is a React error boundary. It enhances the capability of the first error boundary above by allowing for logging errors. Apart from the UI message, developers can also have a error.logging using this to analyze the errors.
Conclusion In this blog, we have seen the various lifecycle methods and their usage, both in old and new version. Below is the flowchart image of both the lifecycle structure (old and new).
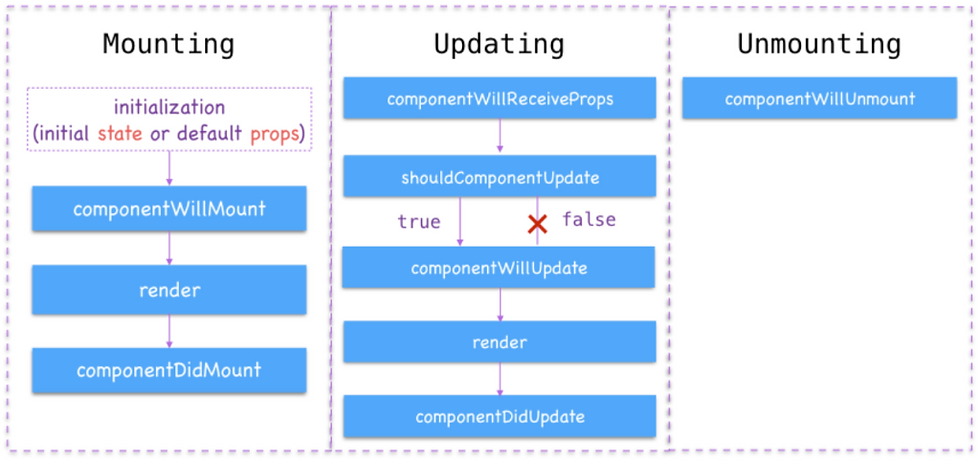
Prior to react 16 --- https://rangle.github.io/react-training/react-lifecycles/
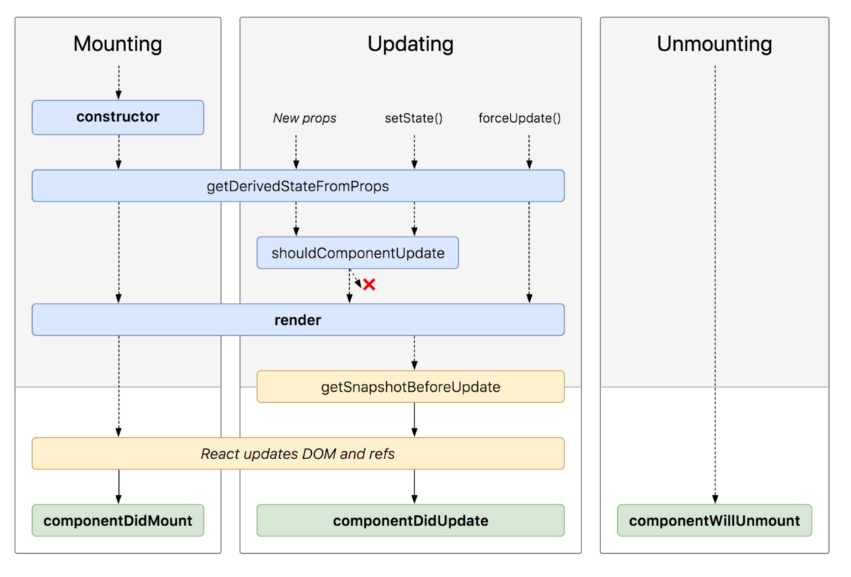
After React 16 ---- https://github.com/wojtekmaj/react-lifecycle-methods-diagram
Comments