What are React Events? Which event handler to use?
- Titash Roy
- Jul 5, 2020
- 2 min read
Updated: Jul 28, 2020
Introduction Events are nothing but user actions performed on DOM elements. Just like HTML, React also, allows actions based on user events. React has the same events as HTML: click, change, mouseover etc. But there is a difference in syntax in React JSX. 1. The action is written in camel case, rather than in lowercase. 2. In React, we pass a function as an event handler rather than a string.
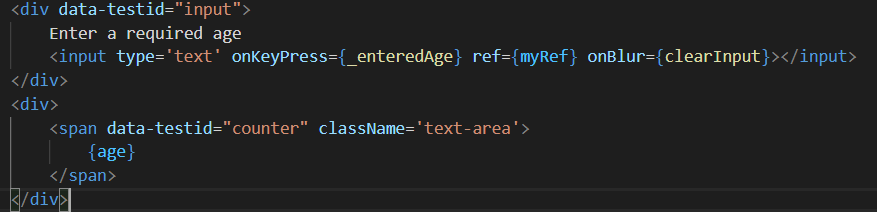
Fig: onKeyPress is the event
3. In contrast to HTML, for preventing the default behavior of an event, we need to add the below snippet.
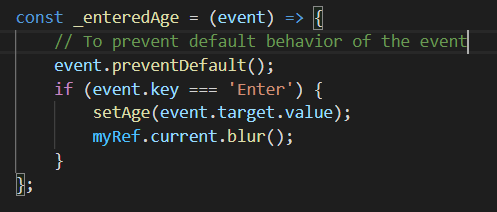
Event Handlers in components There are several ways to handle events in components. One should avoid using anonymous functions as event handlers as it makes the code difficult to read. The best option to write a separate method and call it on events. Different ways to do that are as follows. 1. Binding the method with this keyword: In case the event handler is used to update the class state, we need to bind it with the this instance, failing which, the event handler method, would loose its hold on the class state. This binding should be done in the constructor. Below is a snippet. In the snippet, _increaseAge is the event handler and it is bound inside the constructor method.
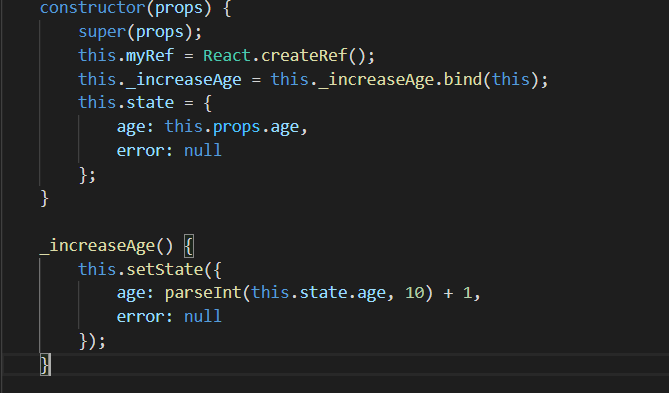
2. Binding the handler in the event callback itself: Yet another way to bind the event handler is along with the event itself associated with the DOM element. Below is a snippet. In the below snippet, _decreaseAge, is bound in the event call itself and not in constructor.
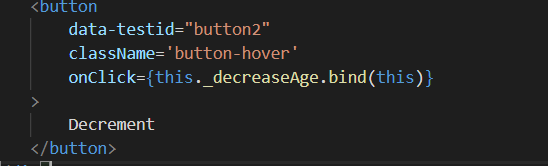
NOTE: With normal functions, the this keyword should be bound with bind to the class instance, as in normal functions, this is not defined by default during its creation. 3. Arrow function: With the introduction of ES6, arrow function came into picture. With the arrow function, we need not bind the handler function as mentioned in the above two methods. With arrow functions, this keyword, will always represent the object that defined the arrow function. Below is a snippet for arrow function.
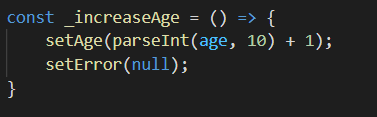
4. Arrow function in the event callback: Just like binding normal function in the callback, we can also use the arrow function declaration in the callback. Below is the snippet.
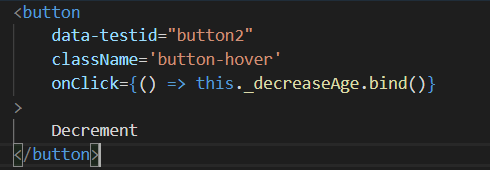
Which is the better way to define an event handler? In the above methods, 2 and 4 are not performance friendly, as everytime the render method is called, there is an unnecessary implicit or explicit binding with the event handler function, though the functions are already defined. Method 1 is invoked only once, during initialization phase, but failing to bind the method in the constructor, can get ugly. Method 3, doesn’t have any performance issues, but is a new concept in ES6. So one should choose the event handler based on the above conditions.
Comments