What are mapStateToProps and mapDispatchToProps?
- Titash Roy
- Aug 2, 2020
- 3 min read
Introduction As discussed in the previous blog, for interacting with the state from redux store, the class components need to have a HOC function, known as connect(). This function, updates the component as per the arguments passed. In this post, we will discuss about two of those arguments: mapStateToProps and mapDispatchToProps. Connect: mapStateToProps This argument is used to extract data from the store. It is the first argument passed in to connect and is used for selecting the part of the data from the store that the connected component needs. In case, we don’t want to pass this argument, it should be denoted with null or undefined, inside the connect function. It takes in the entire store data as input and presents the output as an object as per the component’s needs. It is called every-time the store state changes. MapStateToProps has the following syntax function mapStateToProps(state, ownProps). It can also be denoted as arrow function as below.
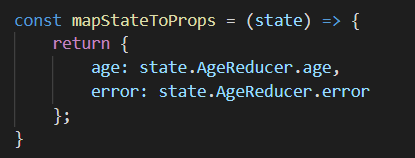
In the above snippet, age is an object, which is generated from the state of the redux store, which contains the reducer values. The first argument should be state, optionally a second argument called ownProps is used and the return is a plain object containing the data that the connected component needs. state: This argument is the state of the redux store and not the store itself. The mapStateToProps, should have this parameter in it. ownProps: This argument is the optional argument. If the component needs the data from its own props to retrieve data from the store, this argument can be used. This argument will contain all of the props given to the wrapper component that was generated by connect. NOTE: mapStateToProps function should be a Pure function. It should not be used to trigger asynchronous behavior like AJAX calls for data fetching, and the functions should not be declared as async. Connect: mapDispatchToProps This is the second argument passed in to connect and is used for dispatching actions to the store. Dispatch is a function of the Redux store. We can call store.dispatch to dispatch an action, but for this to work, we would need to import store into our component, which is not advisable. With React Redux, the components never access the store directly – connect does it for us. With React-Redux we get two ways to dispatch actions: # By default, a connected component receives props.dispatch and can dispatch actions by wrapping the action with the props.dispatch(). In the below snippet, increment_age is the action defined in the actions class.

# connect can accept an argument called mapDispatchToProps, which lets us create object that has functions, which dispatch actions when called, and pass this object as props to our component. In the below snippet, increment is a function present in the returned object, that dispatches the action increment_age and this function is called on the _increaseAge event.
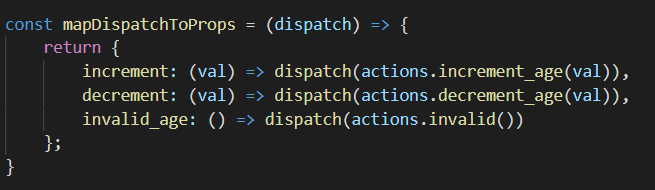
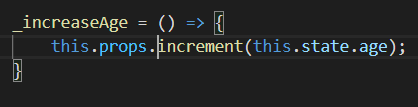
MapDispatchToProps function can take two parameters, dispatch as the first parameter and the ownProps passed to the connected component as the second parameter, and will be re-invoked whenever the connected component receives new props. This means, instead of re-binding new props to action dispatchers upon component re-rendering, we may do so when our component’s props change. There are two prominent ways to write the mapDispatchToProps function. # One is defining it as a function as displayed in the above snippet, which takes dispatch and ownProps(optional) as arguments and returns the object as a prop to the connected component. # By using bindActionCreators. It is a dependency that comes with the Redux module. It is used to bind the action-creators with the dispatch from store. Below is a snippet for the same.
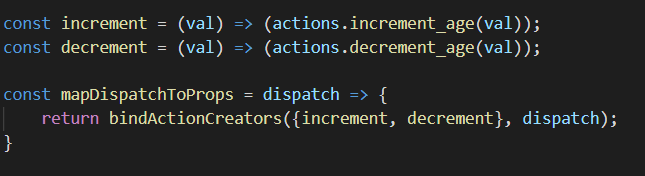
Summary In this blog, we have learnt about the two most widely used arguments inside the connect function. The source code for the same can be found in https://github.com/Titashr/my_react_app.git
Comments